Understanding Data Annotations in Software Development
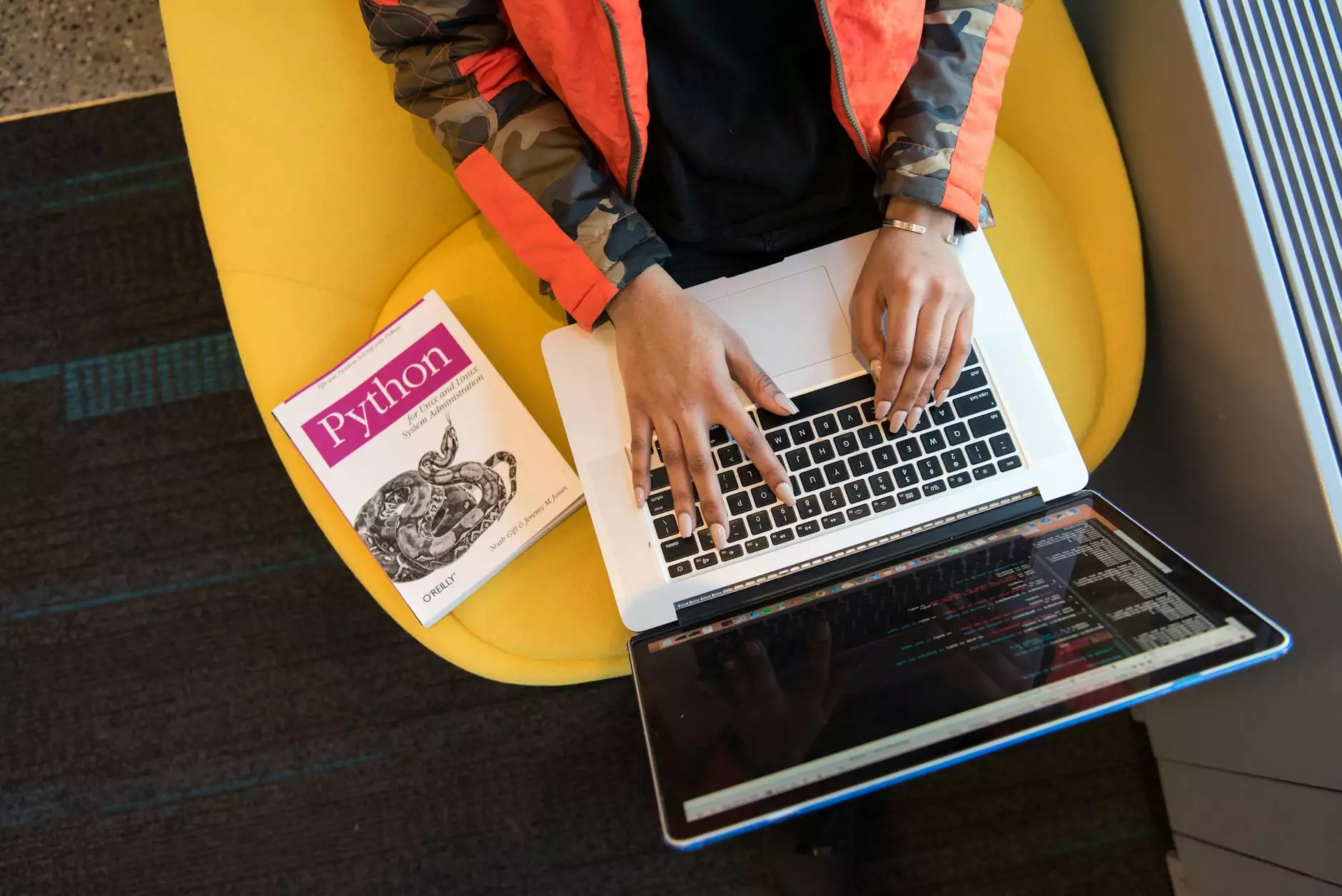
Data annotations are a powerful feature in software development that allow developers to add metadata to classes and properties. This metadata is crucial for defining validation rules, display information, and other characteristics that can enhance the usability and maintainability of applications. In this comprehensive article, we'll explore the significance of data annotations, their practical applications, and how they can significantly improve your software development process.
What Are Data Annotations?
At its core, data annotations are attributes applied to data models in an application, primarily in the .NET framework, but also in many other languages and frameworks. These attributes include information about how the data should be validated, displayed, or processed. They serve as a convenient way to enforce rules directly within the data model, rather than scattering logic throughout the codebase.
Why Are Data Annotations Important?
The use of data annotations is significant for several reasons:
- Simplified Validation: They provide a straightforward way to implement validation rules, minimizing the amount of code needed to ensure that user input meets specific criteria.
- Improved Readability: By keeping validation rules close to the data model, developers can quickly understand how data should be handled without having to search through a plethora of validation logic.
- Consistent User Experience: They help enforce a uniform approach to validation and display across the application, leading to a more cohesive user experience.
- Enhanced Maintenance: When changes to validation rules or display formats are needed, data annotations allow for quick updates without the risk of unintentionally breaking other parts of the code.
Common Types of Data Annotations
There are various types of data annotations that developers can utilize to convey specific attributes and validation guidelines. Here are some of the most common:
1. Required Attribute
The [Required] annotation specifies that a particular data field must have a value. This is particularly important for fields like usernames and passwords where empty values are not acceptable.
2. String Length Attribute
The [StringLength] annotation restricts the number of characters a user can input. This is useful in scenarios where database design limits the size of a field.
3. Range Attribute
The [Range] annotation ensures that a numerical value falls within a specified range, such as age or price ranges.
4. Regular Expression Attribute
The [RegularExpression] annotation enables developers to specify a pattern that the input must match, making it handy for validating formats, such as email addresses or phone numbers.
5. Custom Validation Attribute
For complex validation requirements, developers can create custom annotations by inheriting from the ValidationAttribute class, providing tailored validation logic that meets specific needs.
Implementing Data Annotations
Implementing data annotations in your application is straightforward. Below is a simple example demonstrating how to apply them within a model class in a .NET application:
public class User { [Required(ErrorMessage = "Username is required.")] [StringLength(50, ErrorMessage = "Username cannot be longer than 50 characters.")] public string Username { get; set; } [Required(ErrorMessage = "Email is required.")] [EmailAddress(ErrorMessage = "Invalid Email Address.")] public string Email { get; set; } [Range(1, 120, ErrorMessage = "Age must be between 1 and 120.")] public int Age { get; set; } }Advantages of Using Data Annotations
Utilizing data annotations can significantly boost not only your productivity but also the quality of your software. Here are some of the compelling advantages:
- Less Boilerplate Code: Since validation logic is defined within the model, there's less need for additional code in controllers or view models.
- Automatic Client-side Validation: Many frameworks automatically generate client-side validation scripts based on server-side data annotations, leading to better performance and user experience.
- Clear Documentation: By leveraging data annotations, the model serves as its own documentation, providing immediate insight into how data should be treated.
- Integration with MVC Frameworks: Popular MVC frameworks, such as ASP.NET MVC, utilize data annotations for model binding and validation, which makes its use almost ubiquitous in modern web applications.
Best Practices for Data Annotations
While data annotations are powerful, their effectiveness can be greatly enhanced by following some best practices:
1. Keep It Simple
Use data annotations for common validation scenarios. For more complex logic that can't be captured through annotations, consider implementing validation in separate classes or using custom validation attributes.
2. Use Clear and Concise Error Messages
When defining error messages, ensure that they are understandable and provide actionable advice for users. This will help users correct their input more efficiently.
3. Consistency is Key
Maintain consistency across your application. If you decide to use specific data annotations, try to apply them uniformly to similar data fields across different models.
4. Perform Unit Testing
Regularly test your data annotations to ensure that they are functioning as expected. Identify edge cases that may lead to unexpected validation errors and handle them appropriately.
Conclusion
Data annotations are a fundamental aspect of modern software development that can greatly simplify the process of model validation and ensure that applications are robust and user-friendly. By strategically implementing data annotations in your software projects, you will not only enhance the overall quality of your code but also improve the user experience.
Learn More About Data Annotations
Interested in incorporating data annotations into your next project? At Keymakr.com, we offer extensive resources and professional advice on software development practices, including the effective use of data annotations. Contact us today for more information!